Commit d84748
2024-11-17 13:36:49 Qwas: Save c++入门/dev/null .. cpp/c++\345\205\245\351\227\250.md | |
@@ 0,0 1,83 @@ | |
+ | # c++入门 |
+ | |
+ | - `endl` 用于向流的末尾部位加入换行符 |
+ | - `cout` 代表显示器 |
+ | - `cout << x` 操作就相当于把x的值输出到显示器 |
+ | |
+ | |
+ | ## 输入 Hello World |
+ | |
+ | ```cpp |
+ | # include<iostream> |
+ | using namespace std; |
+ | int main() { |
+ | cout <<"Hello World!\n"; |
+ | } |
+ | ``` |
+ | |
+ | ## 输入输出 |
+ | |
+ | ```cpp |
+ | #include <iostream> |
+ | |
+ | using namespace std; |
+ | |
+ | int main() { |
+ | int a, b, result; |
+ | cout << "please input two number:\n"; |
+ | cin >> a >> b; |
+ | result = 3 * a - 2 * b + 1; |
+ | cout << "result is " << result << endl; |
+ | } |
+ | ``` |
+ | 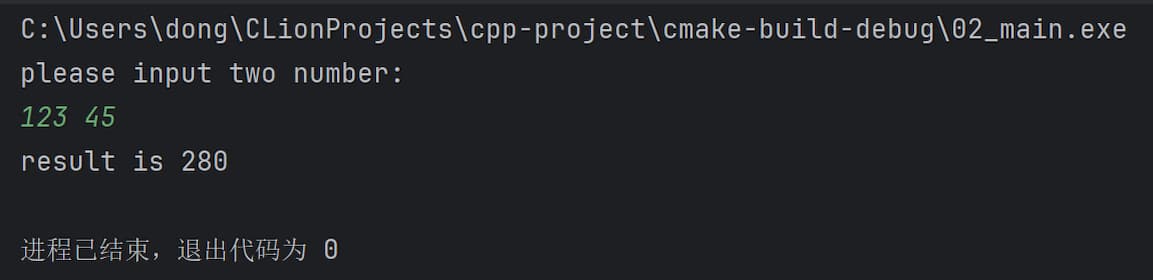 |
+ | ## 使用函数 |
+ | |
+ | ```cpp |
+ | #include<iostream> |
+ | #include<cmath> |
+ | using namespace std; |
+ | |
+ | double max(double x, double y); |
+ | |
+ | int main() { |
+ | double a, b, c; |
+ | cout << "input two number:\n"; |
+ | cin >> a >> b; |
+ | c = max(a, b); |
+ | cout << "maximum=" << c << "\n"; |
+ | cout << "the squart of maximum=" << sqrt(c); |
+ | } |
+ | |
+ | double max(double x, double y) { |
+ | if (x > y) |
+ | return x; |
+ | else |
+ | return y; |
+ | } |
+ | |
+ | ``` |
+ | 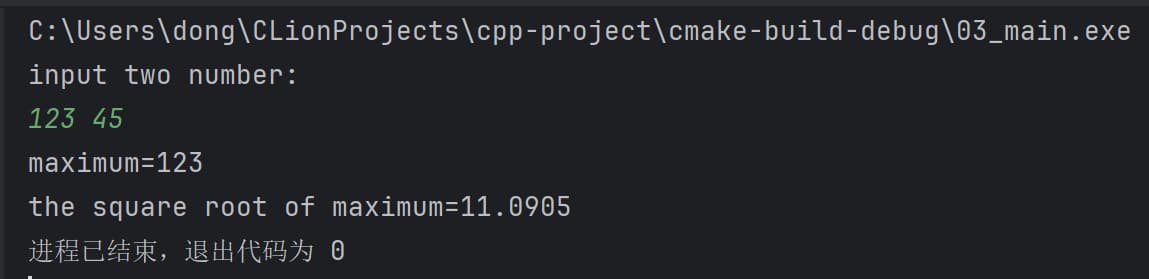 |
+ | |
+ | ## 函数定义包含函数声明 |
+ | |
+ | ```cpp |
+ | #include<iostream> |
+ | #include<cmath> |
+ | using namespace std; |
+ | |
+ | double max(double x, double y) { // 既是函数定义又是函数声明 |
+ | if (x > y) |
+ | return x; |
+ | else |
+ | return y; |
+ | } |
+ | |
+ | int main() { |
+ | double a, b, c; |
+ | cout << "input two numbers:\n"; |
+ | cin >> a >> b; |
+ | c = max(a, b); |
+ | cout << "the squart of maximum=" << sqrt(c); |
+ | } |
+ | ``` |